- Courses
- Python Full Stack Development
Python Full Stack Development
About This Course
This Python certification course will give you hands-on development experience and prepare you for an exciting career as a professional Python programmer.
- 5 Months Course
- 2 Months Internship
- Certificate
Tools and Programs You Will Learn
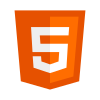
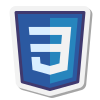
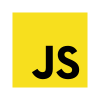
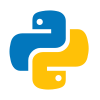
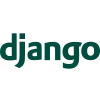
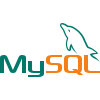
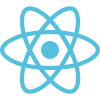
Key Points
- Expert Instructors to Learn from industry leading professionals.
- Flexible Learning to Study anytime anywhere at your pace
- Interactive Courses to Engaging materials for the best experience
- Affordable Excellence and High-quality education at a reasonable cost
Scope
Software Engineer | front-end software / web developer | Python developer or programmer | DevOps engineer.
Curriculum
The Rootsys International has been exclusively founded to groom young leaders in the art of savoir-vivre or social etiquette, Our curriculum and programmes for complete personality enhancement are designed to be a life changing experience.
Item #1
Item #2
Item #3
FAQs
Helping You Understand Rootsys Better
What modes of training does rootsys international provide ?
At Rootsys, we conduct both online and offline training programs to ensure that there is a balanced interaction with the students. It is definitely a lot of commitment that we have taken to make Rootsys International the No 1 software training institute in Malappuram that actually cares for your career growth. ONLINE /OFFLINE
Does Rootsys International Provide Placements Assistance ?
Yes. Rootsys has tied up with many key software firms and we train our students for industry behavior with full assistance until they get the job. We tune them accordingly to face job interviews and new opportunities with confidence
Does Rootsys provide a 100% Placement Guarantee ?
Yes, Rootsys provides a 100% placement guarantee and we do all that it takes to get you a job. We will provide you assistance for getting a job including resume review, interview preparation and mock interview and we train you better to easily convert any opportunity into a job offer.
How do I enroll in a course?
Simply sign up for an account, choose a course from our catalog, and follow the enrollment instructions.
Do I receive a certificate after completing a course?
Yes, all completed courses come with a certificate that you can add to your resume or LinkedIn profile.
More Questions?
Schedule a call with our educational advisor, or email us at info@rootsysinternational.com